
Decorator Design Pattern in Python
Decorators are widely used in Python and an extensively written topic. In this blog we will see what is decorator design pattern, when to use use it and how to code it.
To learn about basics of Python decorators, refer to the following article
By definition, decorator pattern attaches additional responsibilities to an object dynamically. In other words decorator pattern creates wrappers over an object such that object gets additional functionality defined by the wrappers.
Let’s take an example to understand decorator pattern. Suppose we have to develop an app that lets users create stories in different social media apps like Facebook, Instagram, Snapchat, Linkedin, Whatsapp etc. To implement this we can have a story creation function defined in the base class so that every social media platform’s child class can inherit the functionality from the base class.
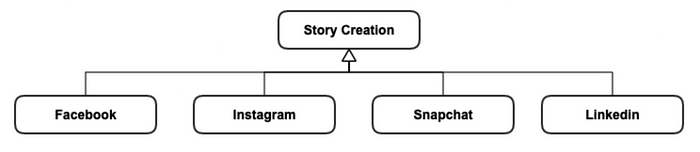
Users love this app and want an additional feature to be able to create stories on multiple social media platforms at the same time. A simple solution for this is to create a story creation class for every combination of social media platforms, i.e. a class that creates story in Facebook and Instagram, another class that creates stories in Instagram, Facebook and Snapchat, and so on. This way each class is doing a very specific functionality. However, this leads to Python class explosion.

To avoid Python class explosion problem, we can use decorator pattern and following is the UML diagram which represents it. We have an interface Story and class ConcreteStory implements interface Story.
As per the definition of the decorator pattern, additional functionality to the ConcreteStory class object can be added by StoryDecorator class.
StoryDecorator can have multiple child classes. It is interesting to notice that StoryDecorator has both “is-a” and “has-a” relation with class Story.

Following is the Python code for decorator pattern. Classes Facebook, Instagram, Snapchat, Whatsapp and Linkedin define the story creation in the corresponding social media platforms. Functions show_off and professional wrap the ConcreteStory with different social media classes objects to add additional functionalities.
Following is the output of the code
Activating show off mode. Let's flood it on the platforms!
Creating story on ...
- Snapchat
- FacebookLet's keep it professional
Creating story on ...
Thank you for reading the article. Following is the link other design pattern articles,
More content at plainenglish.io